1. this 키워드
- 객체 자신을 가리키는 자바스크립트 키워드
- DOM 객체에서 객체 자신을 가리키는 용도로 사용
예) <div> 태그 자신의 배경을 orange 색으로 변경
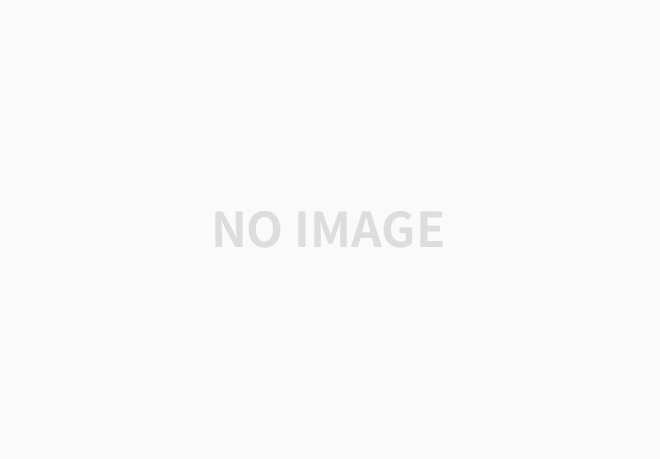
예) 버튼이 클릭되면 자신의 배경색을 orange로 변경
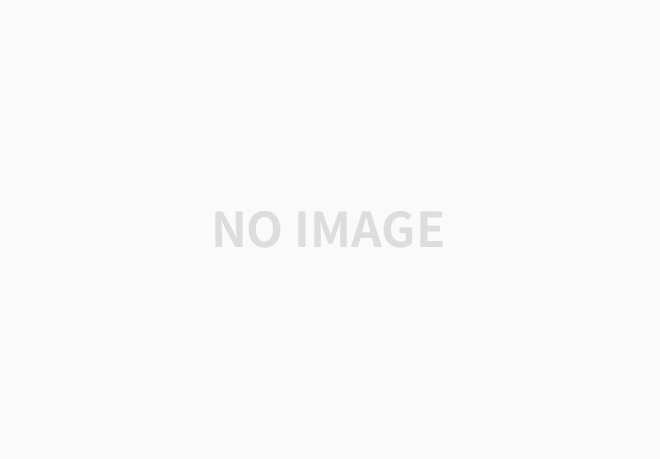
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>this</title>
<script>
function change(obj, size, color){
obj.style.color = color;
obj.style.fontSize = size;
}
function change2(){
t = document.getElementById("n1");
t.style.fontSize = "50px"
}
</script>
</head>
<body>
<h3>this의 활용</h3><hr>
<button onclick="change(this,'30px','#FFA7A7')">버튼1</button>
<button onclick="change(this,'40px','#B2CCFF')">버튼2</button>
<p id="n1" onclick="change2()">여기 클릭</p>
<p id="n1" onclick="change2()">여기 클릭1</p><!--id는 같은 값은 사용하지 않는다. 이 경우 윗 줄만 효과 받음-->
</body>
</html>
http://colorscripter.com/info#e" target="_blank" style="color:#4f4f4ftext-decoration:none">Colored by Color Scripter
|
http://colorscripter.com/info#e" target="_blank" style="text-decoration:none;color:white">cs |
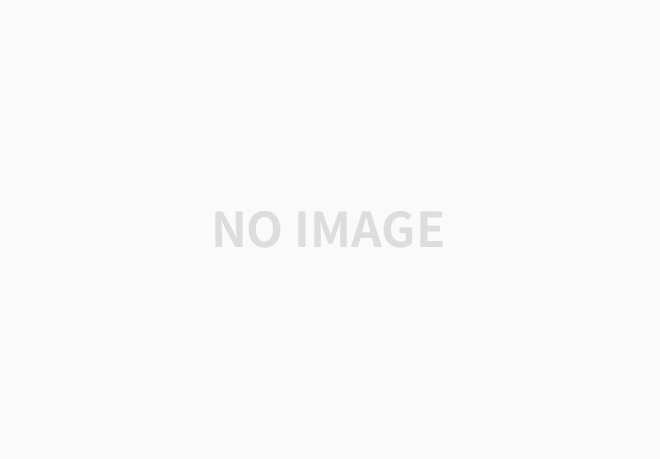
2. document 객체
- HTML 문서 전체를 대변하는 객체
프로퍼티 - HTML 문서의 전반적인 속성 내포
메소드 - DOM 객체 검색, DOM 객체 생성, HTML 문서 전반적 제어
- DOM 객체를 접근하는 경로의 시작점
- DOM 트리의 최상위 객체
브라우저는 HTML 문서 로드 전, document 객체를 먼저 생성
document 객체를 뿌리로 하여 DOM 트리 생성
document 객체 접근
- window.document 또는 document 이름으로 접근
- document 객체는 DOM 객체가 아님
연결된 스타일 시트가 없음
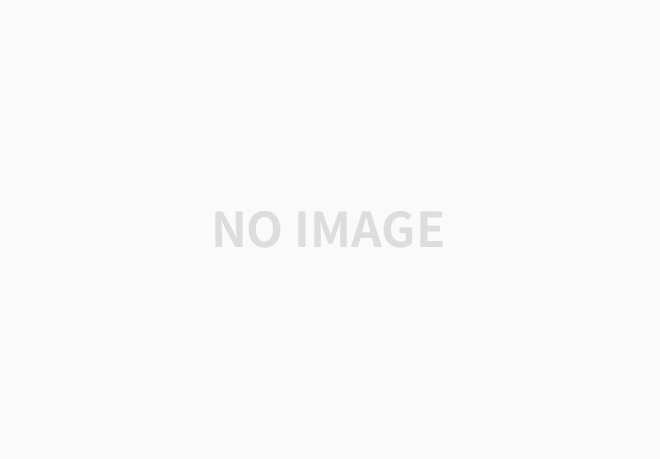
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
|
<!DOCTYPE html>
<html lang="en">
<head id="My Head">
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document 객체 주요 요소값</title><!--document : 브라우저 자체를 가르킨다.-->
<script>
var text = "문서 로딩 중일때 readyState = " + document.readyState + "\n";
</script>
</head><!--onload : 팝업 띄울 때 자주 사용-->
<body onload="printProperties()" style="background-color: lemonchiffon; color: slategray; direction: rtl;">
<div>이곳은 div 영역입니다.</div>
<input id = "input" value="여기 포커스가 있습니다.">
<script>
function printProperties(){
document.getElementById("input").focus();
text+="1.location =" + document.location + "\n";
text+="2.URL =" + document.URL + "\n";
text+="3.title =" + document.title + "\n";
text+="6.domain =" + document.domain + "\n";
text+="7.lastModified =" + document.lastModified + "\n";
text+="8.defaultView =" + document.defaultView + "\n";
text+="9. 문서의 로드 완료 후 readyState =" + document.readyState + "\n";
text+="10.documentElement의 태그이름 =" + document.documentElement.tagName + "\n";
alert(text);
}
</script>
</body>
</html>
http://colorscripter.com/info#e" target="_blank" style="color:#4f4f4ftext-decoration:none">Colored by Color Scripter
|
http://colorscripter.com/info#e" target="_blank" style="text-decoration:none;color:white">cs |
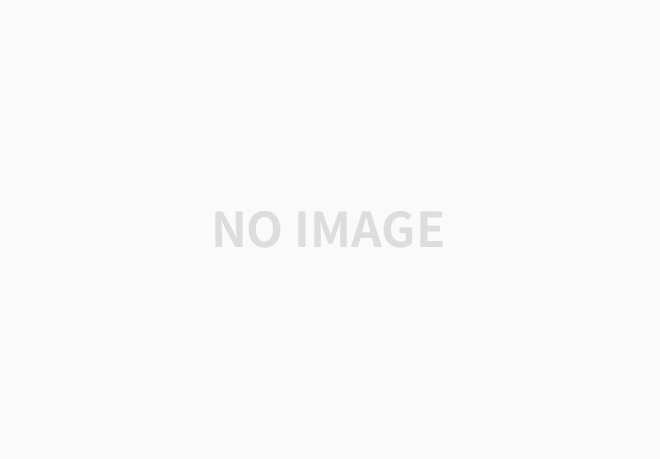
3. DOM 트리에서 DOM 객체 찾기
태그 이름으로 찾기
- document.getElementsByTagName() : 태그 이름이 같은 모든 DOM 객체들을 찾아 컬렉션 리턴
예) <div> 태그의 모든 DOM 객체 찾기
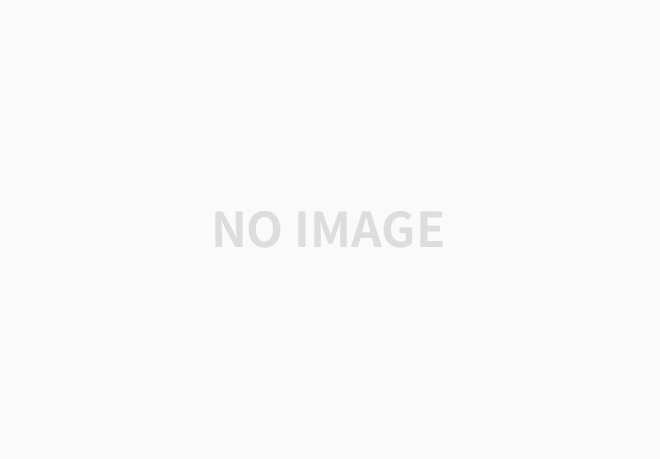
class 속성으로 찾기
- document.getElementsByClassName() : class 속성이 같은 모든 DOM 객체들을 찾아 컬렉션 리턴
예)
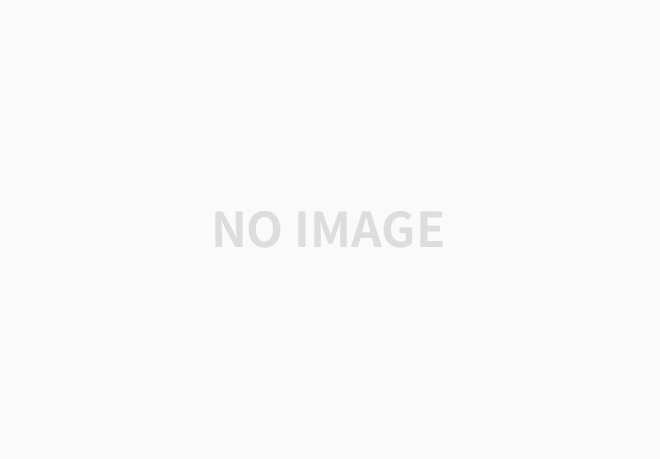
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
|
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script>
function change(){
//태그는 여러개이기 때문에 배열로 리턴한다.
spanArr = document.getElementsByTagName("span");
for(i=0;i<spanArr.length;i++){
span = spanArr[i];
}
}
</script>
</head>
<body>
<h3>내가 좋아하는 과일</h3>
<button onclick="change()">클릭</button>
저는 빨간 <span>사과</span>를 좋아해서
아침마다 한 개씩 먹고 있어요. 운동할 때는 중간 중간에
<span>바나나</span>를 먹지요. 탄수화물 섭취가 빨라
힘이 납니다. 또한 달콤한 향기를 품은 <span>체리</span>와
여름 냄새 물씬 나는 <span>자두</span>를 좋아합니다.
</body>
</html>
http://colorscripter.com/info#e" target="_blank" style="color:#4f4f4ftext-decoration:none">Colored by Color Scripter
|
http://colorscripter.com/info#e" target="_blank" style="text-decoration:none;color:white">cs |
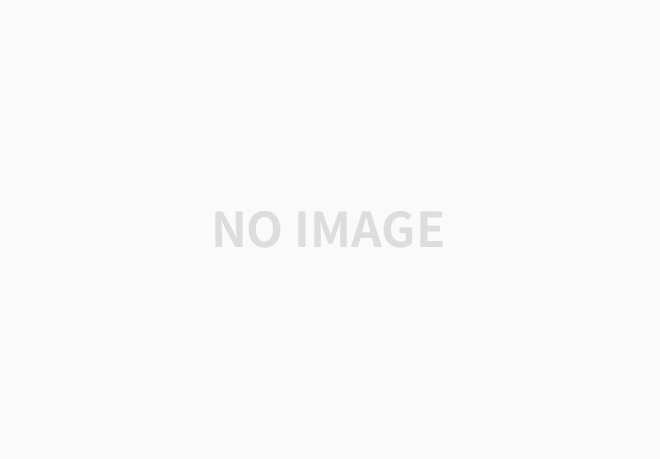
'화면구현' 카테고리의 다른 글
document의 열기와 닫기, open()과 close() (0) | 2020.03.12 |
---|---|
document.write()와 document.writeln() (0) | 2020.03.12 |
HTML DOM(Document Object Model) (0) | 2020.03.11 |
(Javascript) Date 객체, String 객체, Math객체, object 객체 (0) | 2020.03.11 |
(Javascript) 배열 (0) | 2020.03.11 |